when a chatbot meets arduino
If you have worked with IOT projects that integrate with other web apps or any other types of system you probably must be dealing with 10 different languages and frameworks, it’s painful. It also gets chaotic to maintain the code base in different language and repository. In such areas of troubles, Nodejs/javascript gives out some ray of hope. In this post, we will create a chat bot that will be able to communicate with Arduino board and its connected sensor and this entire project will be in Nodejs just one language javascript. Nodejs is chosen because it excels at handles I/O operations.
#Motivation
I have done a couple of chat bot projects, the amazing thing about chat bots is their ease of availability. You don’t have to install another app/plugin on your phone/computer it’s available right in your messenger app just like a jinni waiting to fulfill your wish. And I have also done some side projects on Arduino board, integrating Arduino projects with other systems can be a little tricky, you can have a sensor reading been displayed on a 16x2 LCD, that is cool but it’s can be super cool if it could be delivered in you Messenger app with so suggestions on what action could we do next like if light sensor show reading of 10% or below and its evening then turn on the light or if temperature drops below 10 C the turn on the heater etc. In this post, I have tried to mix the concept of chat bots and Arduino interacting physical world.
#Software required
- Messager platform - Telegram bot API’s are simple to use and there are nice npm modules available which can help us to do quick prototyping.
- Nodejs IOT framework - there are several frameworks available like breakoutjs, firmatajs and johnny-five, but I am going to use johnny-five no specific reason.
- Arduino IDE - Arduino Standard firmware is not going to work with the johnny-five library, we need StardardFirmataPlus Arduino firmware to communicate with the johnny-five library. johnny-five uses Firmata protocol to communicate with the microcontroller, I will get back to this in the later part of the post.
#Hardware required
- Arduino Uno
- Light dependent resistor
- Breadboard
- Jumper wires
Plan of the post is not to create some sophisticated hardware application but just to illustrate the concept, so one sensor is enough for that.
#Basic project setup
We are going to use a couple of Nodejs packages which are :
- johnny-five - IOT framework as discussed before.
- node-telegram-bot-api - High-level wrapper for Telegram bot API’s that takes care of message polling etc, and give nice callback function just to deal with the incoming messages.
To setup the project execute the commands below
1 | mkdir iot-bot && cd iot-bot; |
#Setting up Arduino for johnny-five
To use johnny-five with Arduino we have to upload StandardFirmataPlus firmware to Arduino. So what exactly is StandardFirmataPlus again? In simple terms it’s a general purpose sketch which you don’t program the explicitly sketch to read/write data for particular pins instead, the sketch is programmed to read commands from the host computer to which Arduino is connected and execute it. The command could be writing/reading data to/from Arduino pins. All this is done with a Firmata protocol. It is contrary to the traditional Arduino sketch we write that has all the logic on how to deal with sensor data. Logic is moved to the host computer instead, and a general purpose sketch is uploaded to Arduino that just knows how to deal with I/O pins.
To install StandardFirmataPlus firmware go to File > Examples > Firmata > StandardFirmataPlus it will open up Arduino sketch upload the sketch to Arduino board. To test if everything went right execute the below javascript code on your host computer to which Arduino board is connected and you should get Board is ready printed on your terminal.
1 | var five = require("johnny-five"); |
Arduino Schematic diagram
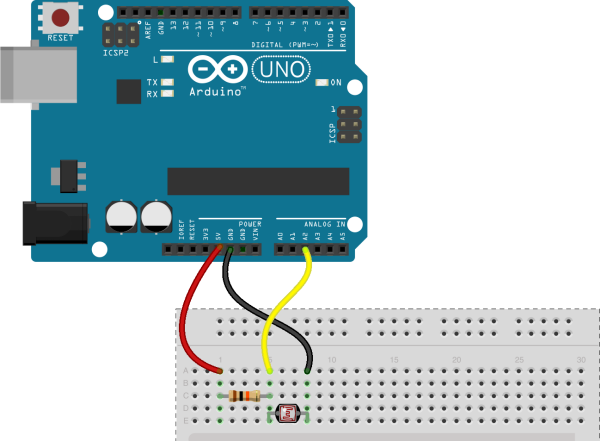
Getting console greeting is not enough, follow the below schematic to connect a light dependent resistor with Arduino board, javascript code below we will read the value of light sensor data from pin A0 and print it on the console.
1 | var five = require("johnny-five"); |
#Setting up Telegram Bot
Till now we have handled the Arduino side of the project, we have to manage to pull the sensor data from Arduino into our Nodejs code, next we have to send it to Telegram messenger. But first, we have to setup Telegram bot. You need Bot token to use Telegram bot API’s you can follow this official post on how to create a bot and get bot token.
Once you have the bot token lets verify that you got things right, execute the javascript code below and fill the token variable with the bot token which you bot from @BotFather.
1 | var TelegramBot = require('node-telegram-bot-api'); |
#Hooking up Arduino with Chat Bot
Now that we are through with different components of the project it time that we hook everything together. The bot will send a notification to you if the value of the light dependent resistor is between 10% to 30 %. This range value (10-30%) was the reading of the sensor when the light was on in my room, so that’s what I am using, you can change it to any other value that suits your environment, threshold value depends lots of factor like the distance between the light source and the sensor so the value might differ for your settings. Another functionality will be reading light sensor value on when asked for, this can be done simply by storing the value of the sensor in the variable when a change event is fired this way we have the last changed value of light sensor, and when user ask’s for sensor reading send the value of the variable.
To receive notifications you have to subscribe by sending /subscribe command to the bot, if you interested to know the value of the sensor right away then type /light command and if you don’t want to receive the notification then type /unsubscribe. Telegram bot platform has commands functionality inbuilt so UI dropdown list shows up so you don’t have to type the whole word. And as you might have noticed commands starts with / which is also part of bot platform. The bot will send push message when the sensor value is within range.
Bot commands summary :
- /light - Read the current value of light sensor
- /subscribe - Subscribe to list of user getting notifications.
- /unsubscribe - Unsubscribe to notifications if not interested.
Below is the code for what we have discussed so far
1 | var five = require("johnny-five"); |
This small project we did it too simple but it was just to illustrate the concept nothing serious project was intended. But now that you have tools you could develop something more sophisticated by mashing up many sensors like temperature sensor, relay circuit etc. You could display Telegram message send by the user to 16x2 LCD display connected to Arduino sitting in your kitchen possibilities are limitless.
#Conclusion
As you saw we implemented chat bot interacting with Arduino in few lines of code. All though the project is very simple, it’s clear that you don’t need to learn another language to program Arduino javascript is enough. Our entire project was in Nodejs but you could use any language of your choice, as there are Firmata protocol client libraries for many languages and also it’s not difficult to make Telegram bot REST API calls from the language of your choice you might even find a GitHub library for that as well. Happy Hacking!